Using Python at the office can be an incredibly powerful way to automate tasks and increase productivity. It can be used to perform a wide range of tasks, including data manipulation, process automation, and performing data analysis.
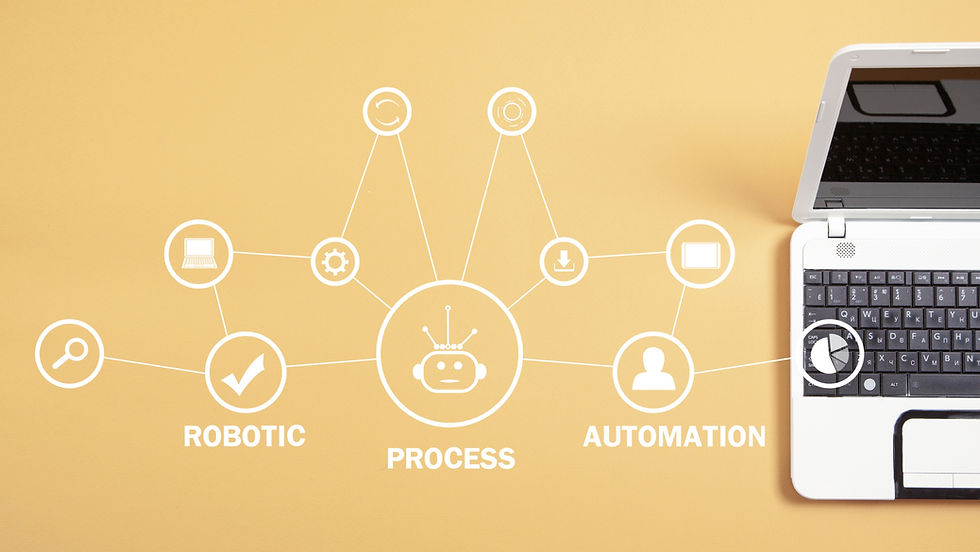
I would like to highlight some of the areas where you might consider using Python to automate tasks and streamline processes.
Use Python to Write Scripts to Automate Repetitive Tasks
If you have tasks that you perform on a regular basis, you can write a Python script to automate such tasks. For example, performing the same task on multiple files or data sets, running a series of data transformation or analysis steps on a regular basis, downloading files with different names from the same or multiple location(s), creating or extracting zip files, and a lot more tasks can be easily automated.
Use Python Libraries to Perform Specific Tasks
There are many Python libraries that can be used to perform specific tasks, such as copy-paste data, generate reports, or interact with websites. By using the below-mentioned libraries, you can save time and effort on tasks that would be frustrating or sometimes difficult to do it manually.
For example,
Python can be used to copy-paste the data from/to a variety of sources/destinations, such as CSV files, Excel sheets, or databases.
For example,
to read a CSV or XLSX file using the Pandas library:
df1 = pd.read_csv("data.csv")
df2 = pd.read_excel("data.xlsx")
to write a CSV or XLSX file using the Pandas library:
df1.to_csv("data.csv")
df2.to_excel("data.xlsx")
these codes are understandable, the first two will source data from CSV and excel spreadsheets into a pandas DataFrame, and the other two will write the DataFrame to the destination spreadsheet. By default, the DataFrame will be written to the first sheet of the destination spreadsheet, and the data will be pasted starting at cell A1.
renaming a series of files using the 'os' library:
import os
directory = "/path/to/files"
files = os.listdir(directory)
for file in files: #iterate through all the files
name, extension = os.path.splitext(file)
new_name = name + "_new" + extension
os.rename(os.path.join(directory, file), os.path.join(directory, new_name))
it will work the same regardless of the number of files and file type.
Python can create zip files. Use the built-in zipfile Python package. Sample code is here to create a zip file by adding multiple files:
import zipfile
zf = zipfile.ZipFile("filex.zip", "w")
zf.write("file1.txt")
zf.write("file2.csv")
zf.write("file3.xlsx")
zf.close()
this was quite simple, right? How much time did it take to create a zip file manually? now automate it!
Python can be used to download a specific file or multiple files (using a for loop) from a URL. The 'urllib' library allows you to download a file from a specified URL and save it to your local system. Here is the code:
import os
import urllib.request
url = "http://www.example.com/path/to/file.txt"
file_path = "/path/to/save/file.txt"
urllib.request.urlretrieve(url, file_path)
Python can be used for sending emails, a common task that is most frequent at the office workplace. Python provides several libraries and tools that can be used to send emails such as 'smtplib' and 'email' (python built-in libraries) that provides function and methods to send emails, provides tools to access different parts of an email (for example, the subject line, body message, and attachments), and powerful functions for encoding and decoding emails in various formats to make integration possible. However, access to the office server may require authentication, such as a username and password which must be taken care of.
Python can be used to generate reports in formats such as PDF or Word documents, often required at the office workplace right? To do this, you will need to use a library such as 'xlsx2pdf' or 'pdfkit' or tools that can be used to convert the Excel file to a PDF or to a Word document.
Python can be used for interacting with websites or APIs to scrape data. And for this, you can use a library called 'requests' to make HTTP requests or 'beautifulsoup' to parse the HTML or XML data. For more information, you can refer to the 'requests' and 'beautifulsoup' library documentation.
Python can be used to perform complex calculations or statistical analysis on large datasets.
For example, the NumPy library provides tools for numerical computing, including functions for basic arithmetic and more advanced operations like linear algebra, random number generation, and statistical analysis. Another awesome library is SciPy, built on top of NumPy and provides additional scientific and technical computing tools such as optimization, interpolation, and signal processing. Pandas library is for handling and manipulating large datasets, and includes functions for reading and writing data from various sources, as well as tools for cleaning and manipulating data and performing basic statistical analysis. Last but not the least, the StatsModels library, for estimating and testing statistical models, can be used for tasks such as regression analysis and time series analysis.
Most interestingly, these libraries can be used together to perform complex calculations and statistical analysis on large datasets, including automation.
Python can also be used to visualize data with charts or graphs. Some popular libraries for data visualization include Matplotlib, Seaborn, Bokeh, and Plotly.
To utilize these libraries, you must install them and import them into your Python script. Afterward, you can utilize their functions and methods to perform these specific tasks.
Using Python to Exchange Data Between Different Software Applications
Python can be used as an interface between different software applications, such as Microsoft Office, to automate tasks and exchange data between them.
For example, Python can be used to extract data from an Excel spreadsheet and use it to generate a report in a Word document or a presentation in PowerPoint. Quite useful right? Another example could be generating a newsletter by sourcing data/information from multiple applications.
By using Python to automate tasks at the office, you can save time and effort, and increase your productivity and efficiency at the office.
Comments