Technical analysis is a popular approach used by traders and investors to make informed decisions in the financial markets. Among the various tools and techniques in technical analysis, support and resistance levels play a crucial role in identifying potential areas of price reversals.
One of the key components of technical analysis is identifying support and resistance levels, which are points on a price chart where traders expect prices to reverse direction. In this Financial Pythonist book, we will delve into the concept of support and resistance levels for equities, exploring their significance and how they can be used to enhance trading strategies using Python.
To identify the support and resistance levels for a specific stock, please refer to Extract Historical Stock Data using API to extract the historical time-series data of the stock for which we want to identify the support and resistance levels.
Python Code:
import yfinance as yf
import pandas as pd
# Specify the stock symbol and the desired date range
stock_symbol = 'AAPL'
start_date = '2023-01-01'
end_date = '2023-06-30'
# Retrieve the historical price data using yfinance
stock_data = yf.download(stock_symbol, start=start_date, end=end_date)
Support and resistance levels are points on a price chart where the price of a security tends to stop moving in a particular direction and reverse its trend. Support levels represent price levels where the demand for a security is strong enough to prevent further price declines, while resistance levels indicate price levels where the supply of a security is strong enough to prevent further price increases. It is worth noting that these levels can be identified by analyzing historical price charts and identifying areas where the price has reversed direction multiple times.
Identifying Support and Resistance Levels Using Python
Assuming that we have already extracted the historical stock data and stored it in a Python dictionary, let us now examine the calculations involved in determining the support and resistance levels.
Data Points
Pivot Point | Support Level 1 | Support Level 2 | Resistance Level 1 | Resistance Level 2
Pivot Point ( PP )
Let me introduce you to the term "Pivot Point." It is a technical analysis indicator used to determine the overall market trend across different time frames. The Pivot Point is calculated based on the previous trading day's price movement, by taking the simple average of the High, Low, and Close prices of the stock.
Pivot Point = (High + Low + Close) / 3
It's important to note that this point is calculated using data from the previous trading day. If the stock price is trading above the Pivot Point on the next trading day, it indicates an ongoing bullish trend in the market. Conversely, if the stock price is trading below the Pivot Point on the next trading day, it indicates an ongoing bearish trend in the market. The Pivot Point serves as a reference point for calculating the support and resistance levels.
Support Level ( S1, S2 )
Support levels are price levels at which buying pressure tends to outweigh selling pressure, preventing the price of an equity from falling further. These levels act as a floor for the price, as demand increases and traders see value in buying the equity. The repeated rebound of the price from support levels indicates market participants' willingness to accumulate the stock, potentially signaling a bullish sentiment. Traders often consider support levels as potential entry points for long positions, placing stop-loss orders just below these levels to limit potential losses.
Support is the level at which traders tend to become bullish. It represents a point where a downtrend is anticipated to reverse due to increased buying interest from traders. In simpler terms, when the stock price falls to a specific level, it triggers increased demand for the stock, resulting in the formation of a support level.
The calculation for support levels is as follows:
Support L1 = ( Pivot Point * 2 ) - High
Support L2 = Pivot Point - ( High - Low )
Resistance Level ( R1, R2 )
Resistance levels, on the other hand, are price levels at which selling pressure tends to outweigh buying pressure, preventing the price of an equity from rising further. These levels act as a ceiling for the price, as supply increases and traders see an opportunity to sell the equity. The price's repeated inability to break through resistance levels suggests market participants' willingness to offload the stock, potentially indicating a bearish sentiment. Traders may consider resistance levels as potential exit points for long positions or opportunities to initiate short positions.
Resistance represents the level at which traders tend to adopt a bearish stance. It is a price level where an ongoing uptrend is expected to reverse due to selling or profit booking by traders. Simply put, when the stock price reaches a certain level of increase, traders tend to take profits, resulting in the formation of a resistance level.
The calculation for resistance levels is as follows:
Resistance L1 = (Pivot Point * 2) - Low
Resistance L2 = Pivot Point + (High - Low)
Python Code:
def calculate_support_resistance(data):
pivot_point = (data['High'] + data['Low'] + data['Close']) / 3
support_l1 = (pivot_point * 2) - data['High']
support_l2 = pivot_point - (data['High'] - data['Low'])
resistance_l1 = (pivot_point * 2) - data['Low']
resistance_l2 = pivot_point + (data['High'] - data['Low'])
return pivot_point, support_l1, support_l2, resistance_l1, resistance_l2
pivot_point, support_l1, support_l2, resistance_l1, resistance_l2 = calculate_support_resistance(stock_data)
# Create a DataFrame to store the calculated levels
levels_data = pd.DataFrame({'Pivot Point': pivot_point,
'Support Level 1': support_l1,
'Support Level 2': support_l2,
'Resistance Level 1': resistance_l1,
'Resistance Level 2': resistance_l2})
print(levels_data)
Result Output:
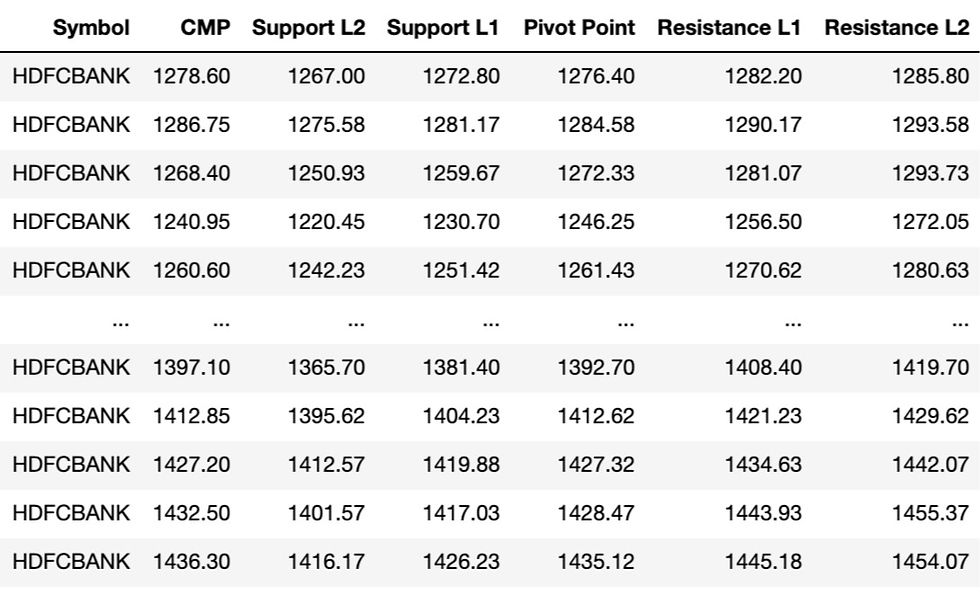
If we were to create a graph using the matplotlib library that displays the Close Price of a stock over a specific period, along with the most recent Support L1, Support L2, Resistance L1, and Resistance L2 levels, the resulting plot would resemble the image depicted below.
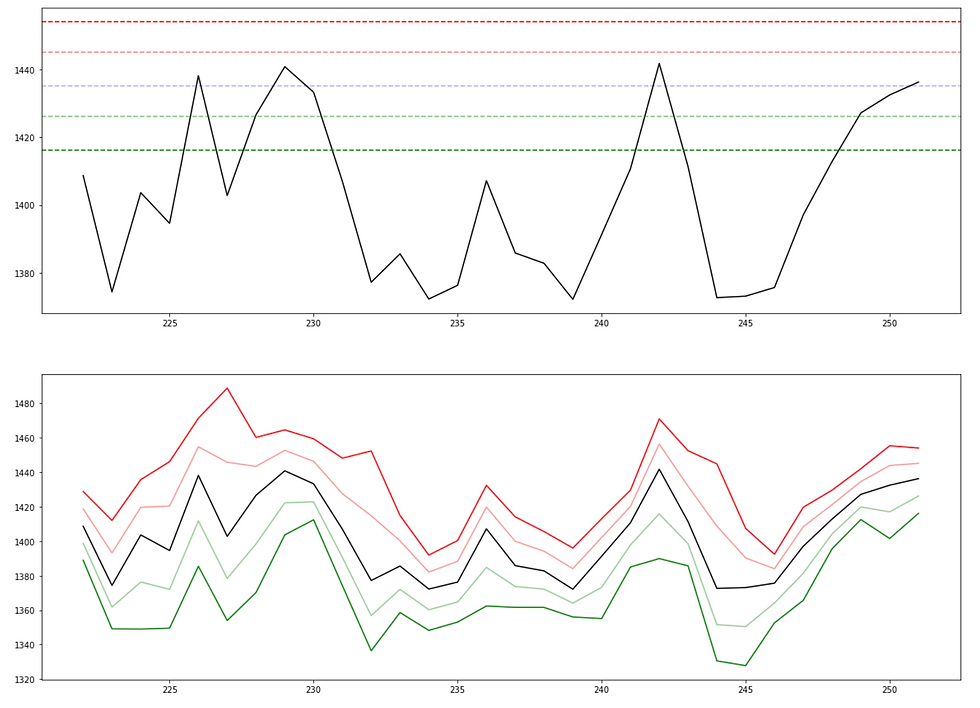
The first plot illustrates the Stock Price with the latest Support and Resistance Levels, while the second plot showcases the Stock Price with moving Support and Resistance Levels.
(Caution: Moving Support and Resistance Levels are lagged by 1 day since we use the previous trading day's data to determine the overall market trend for the next day.)
Since our focus is primarily on the most recent pivot point, support, and resistance levels, we can effortlessly generate a DataFrame that presents all ticker names along with their respective data points for these levels in a row-wise format, as exemplified below.
Result Output:
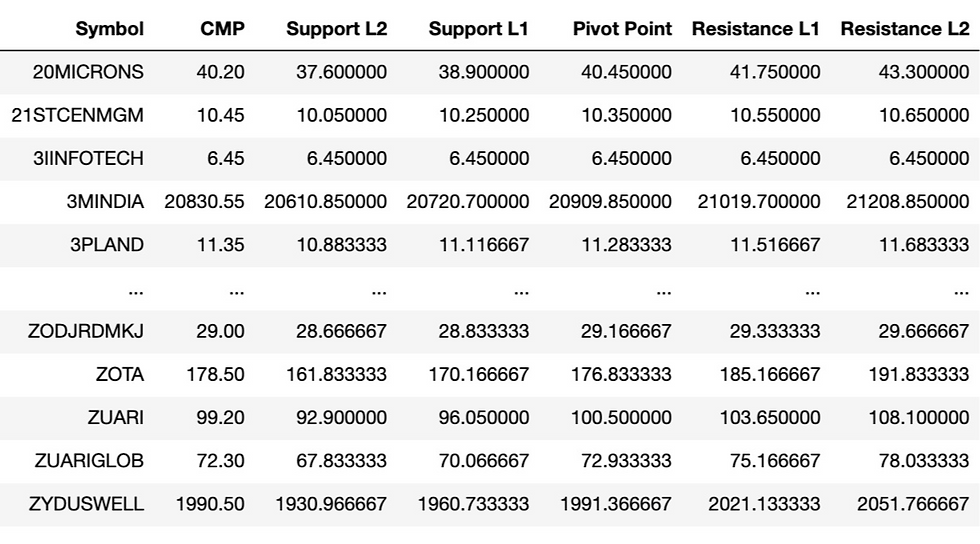
Importance of Support and Resistance Levels
Support and resistance levels are significant because they reflect the collective psychology and behavior of market participants. These levels are derived from historical price data, representing areas where the price has reversed or encountered barriers in the past. As such, they can provide valuable insights into the supply and demand dynamics of an equity, helping traders identify potential turning points and make informed trading decisions.
Support and Resistance Confirmation
The strength of support and resistance levels can vary. A support or resistance level that has been tested multiple times and successfully held is generally considered stronger and more reliable. The more times the price has respected a particular level, the greater the significance attached to it. Traders often look for confirmation of support or resistance levels through factors such as trading volume, chart patterns, and technical indicators to increase the reliability of these levels.
Dynamic Nature of Support and Resistance
It's important to note that support and resistance levels are not fixed or permanent. They can shift and evolve over time as market conditions change. A support level that has been breached may turn into a resistance level, and vice versa. Therefore, traders should continuously monitor price action and adjust their analysis accordingly to stay aligned with the evolving support and resistance levels.
Comments